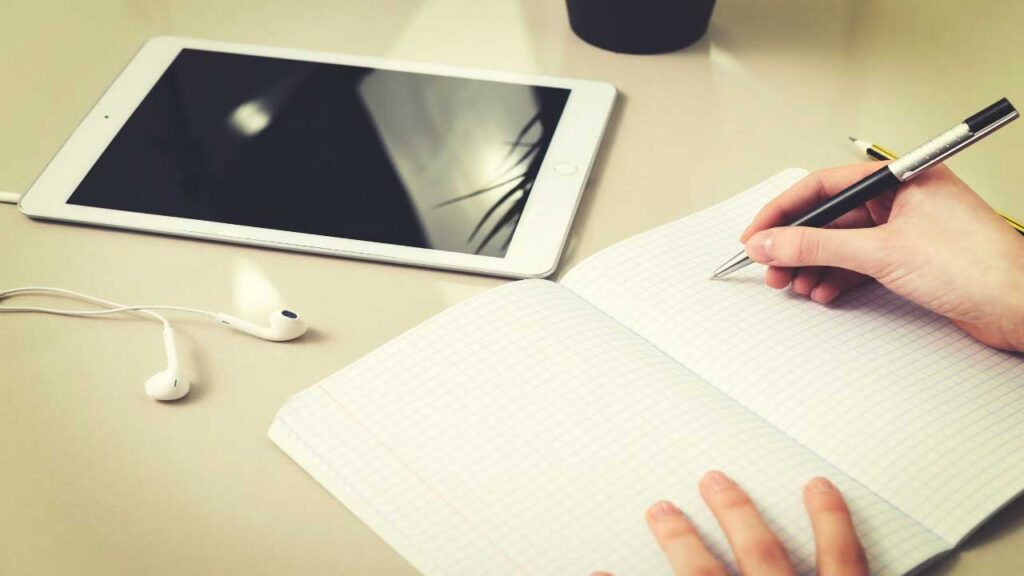
Python Tutorial 7
What Are Data Types (2)
In this tutorial, we will cover Part 2 of Python data types.
Data types will also be covered in more detail in the tutorials on String Manipulation.
Without further ado, let’s get started!
Numeric Data Types
What are numeric data types?
There are 2 main numeric data types, namely:
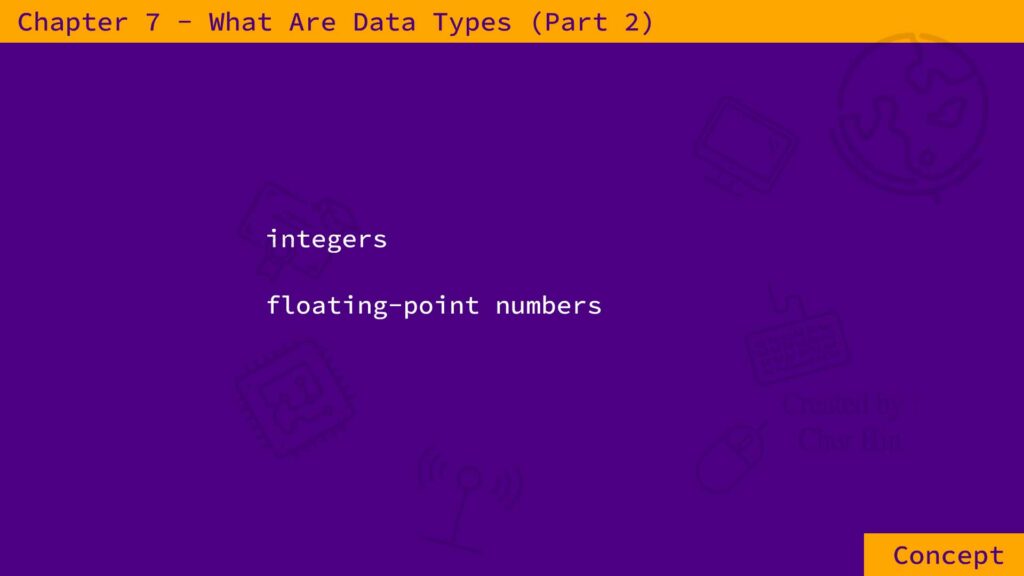
Integers can consist of only the numbers 0–9, and a positive or a negative sign, and with no decimal point.
It is a whole number, which is not a fraction, and can be positive, negative, or zero.
Integers do not have decimal places.
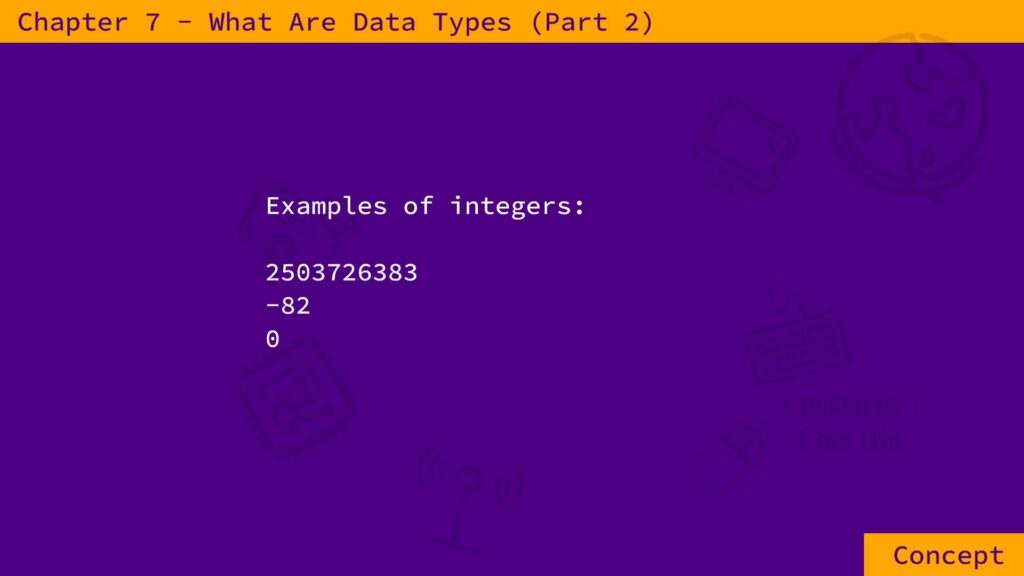
A data type similar to integers is the floating-point numbers which is also a numeric data type.
What differentiates integers from floating-point numbers is the decimal point.
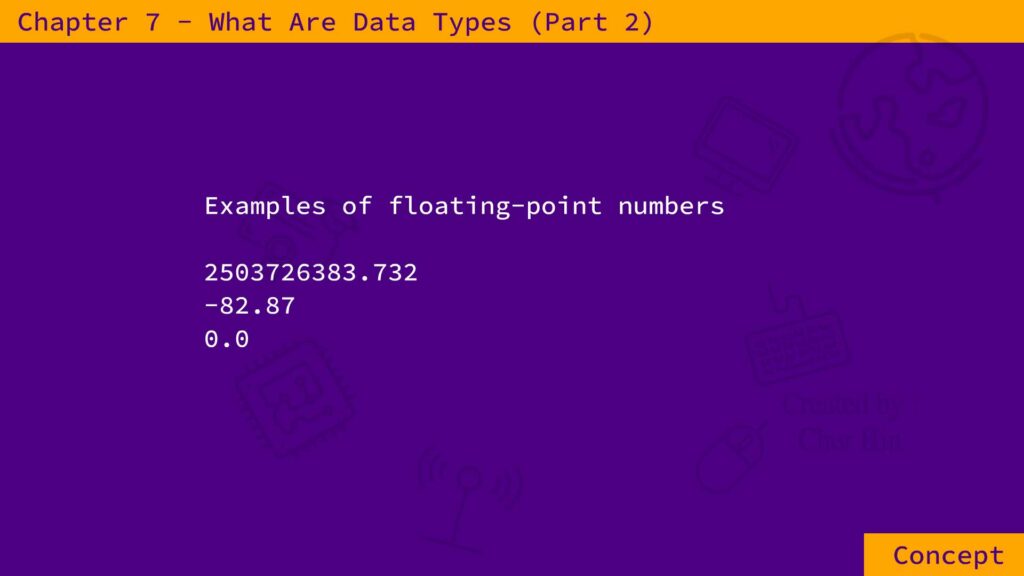
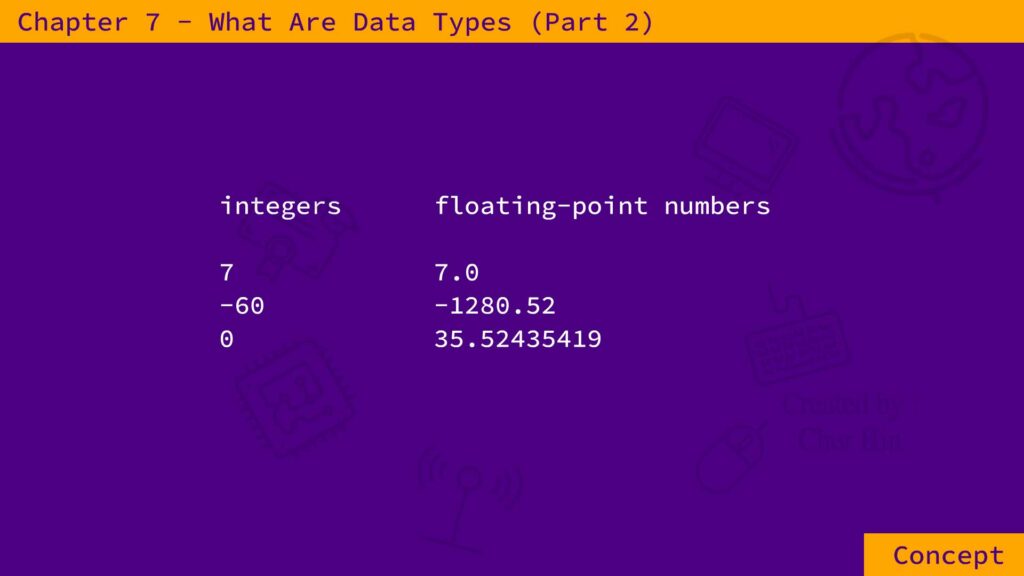
7 is an integer whereas 7.0 is a floating-point number.
-60 is an integer whereas -1280.52 is a floating-point number.
Zero is an integer whereas 35.52435419 is a floating-point number.
If given a choice, use integers instead of floating-point numbers – because the computer processes integers much faster than floating-point numbers.
What is the difference between number equals 2 enclosed within quotation marks, and number equals 2 without the quotation marks?
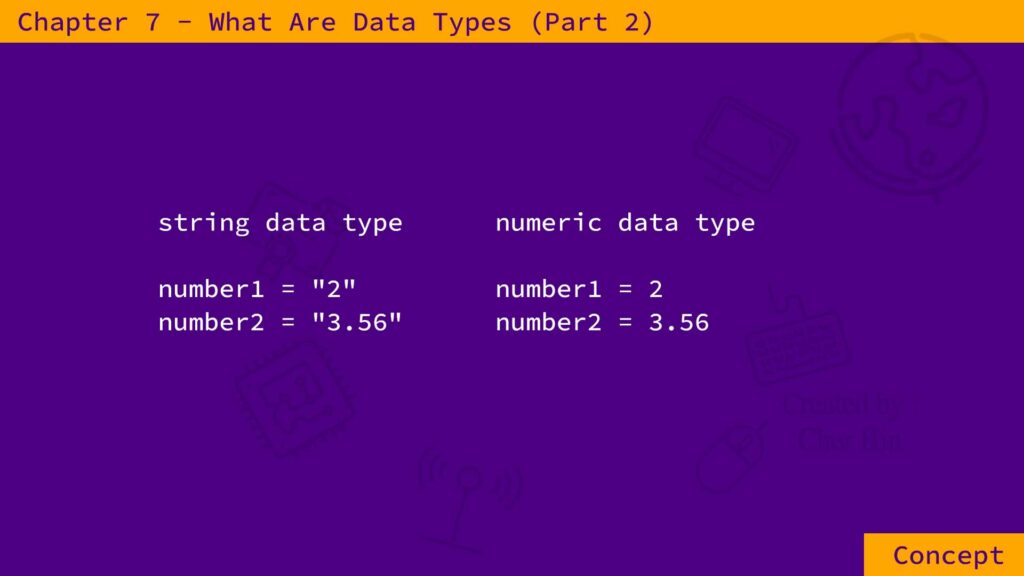
The answer is — that putting numbers within quotation marks makes it a string data type whereas just having the number without any quotation marks makes it a numeric data type.
And commas are not allowed in numeric data types.
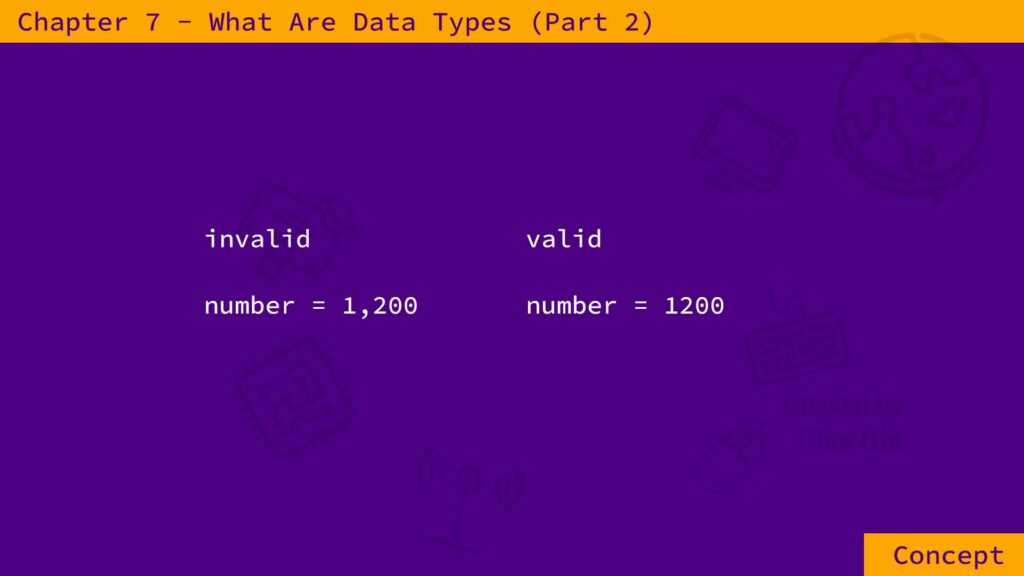
Boolean Data Types
What are Boolean data types?
Booleans have only 2 values — True or False.
Note that they start with an uppercase T and an uppercase F.
Booleans are used in evaluating the True or False of anything passed to it.
The Python built-in function bool() is used to represent the True or False of any argument passed to it.
Only numeric zero value and empty string value passed to the bool() function will return False.
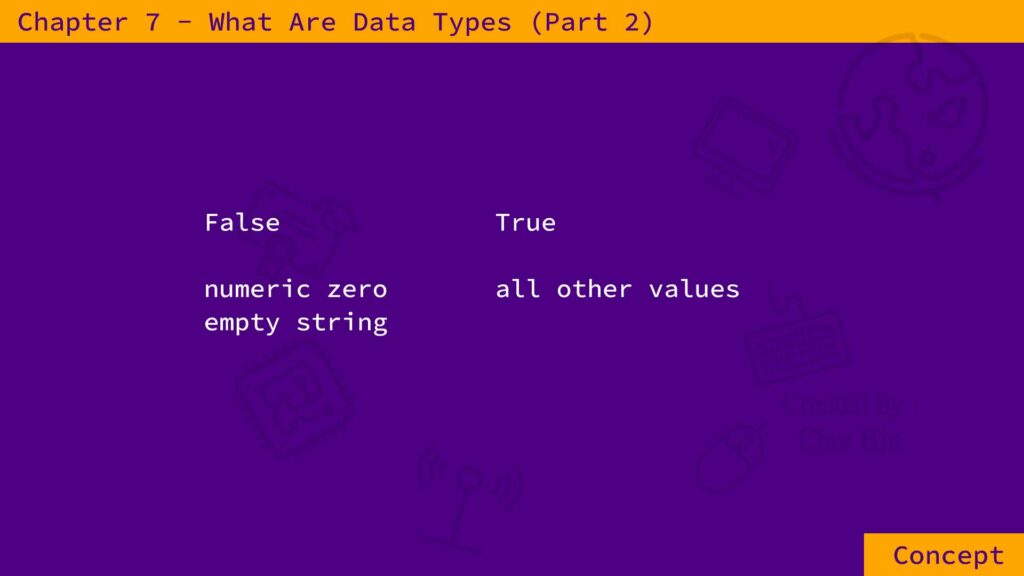
All other values passed to it will return True.
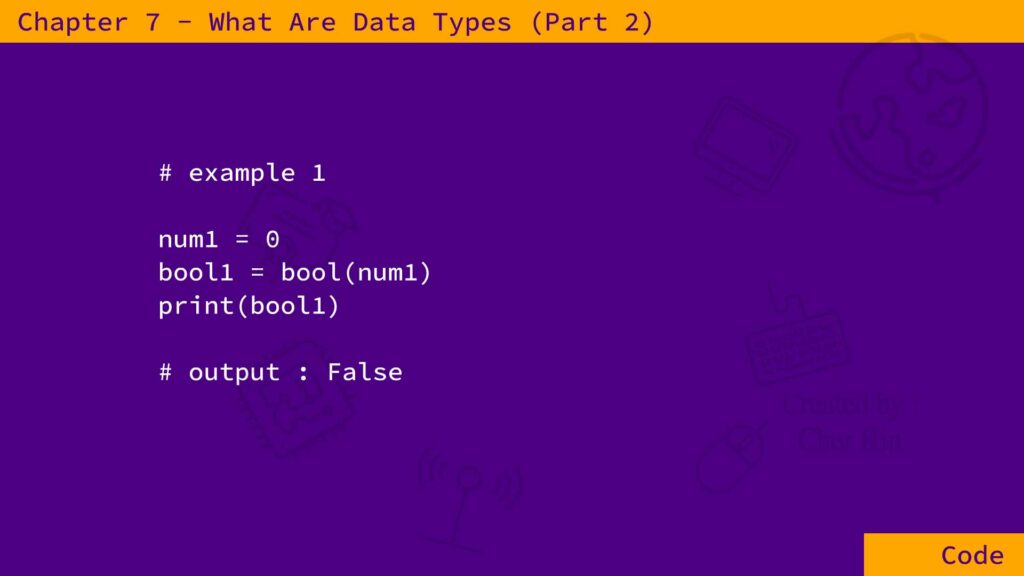
In example 1, the variable num1 is assigned the numeric value of zero.
When we print its boolean value, False will be printed because the boolean of num1, which has a zero numeric value, will return False.
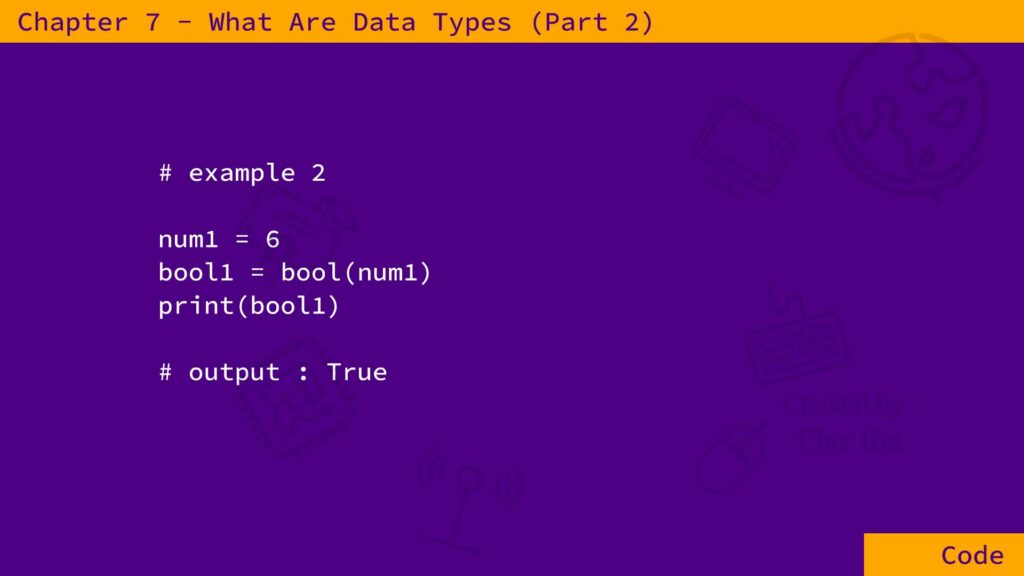
In example 2, the variable num1 is assigned the numeric value of 6.
When we print its boolean value, True will be printed because the boolean of num1, which has a non-zero numeric value, will return True.
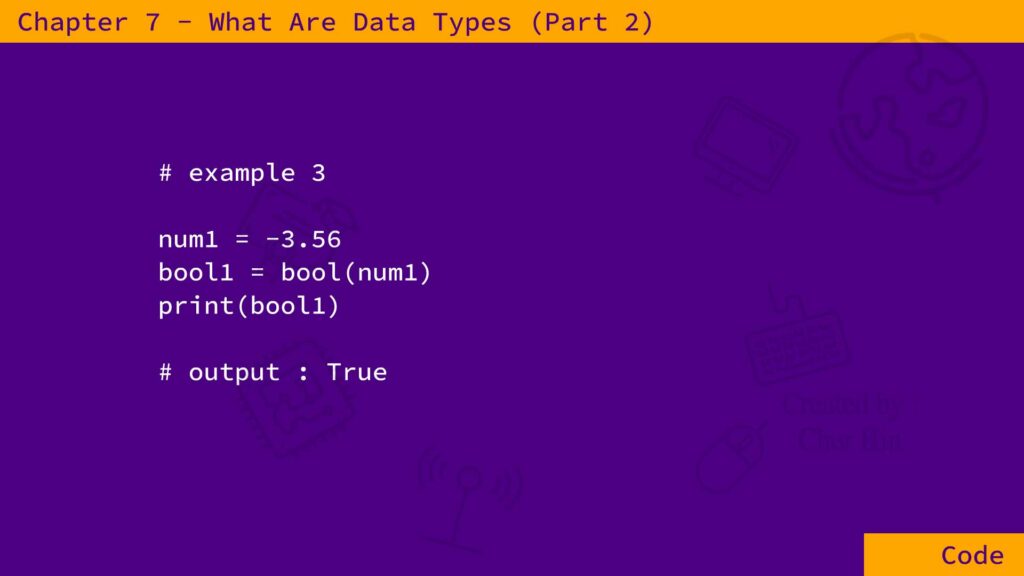
In example 3, the variable num1 is assigned the numeric value of -3.56.
When we print its boolean value, True will be printed because the boolean of num1, which has a non-zero numeric value, will return True.
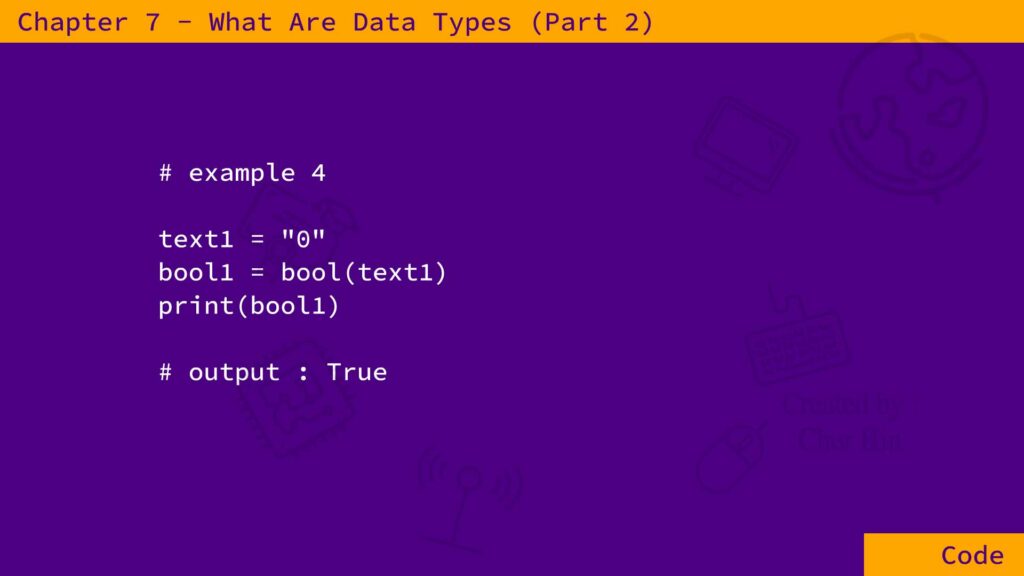
In example 4, the variable text1 is assigned the string value of “0”.
When we print its boolean value, True will be printed because the boolean of text1, which has a non-empty string value, will return True.
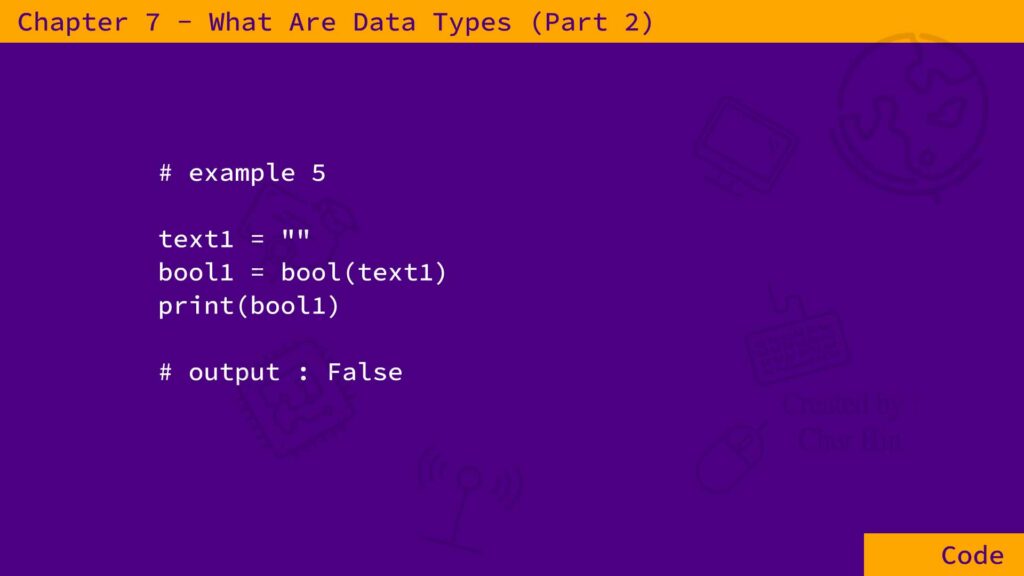
In example 5, the variable text1 is assigned an empty string.
When we print its boolean value, False will be printed because the boolean of text1, which has an empty string value, will return False.
Booleans have so many other uses, including in expressions and string manipulation, which we will come across more in future tutorials.
Python Keyword None
What about the Python keyword None?
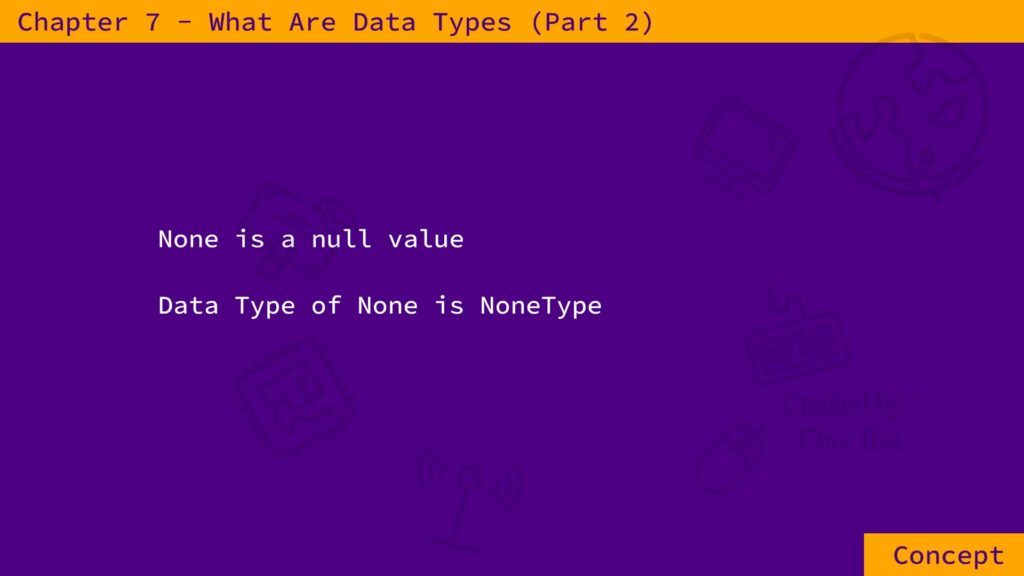
None is a null value and it has its own data type called NoneType.
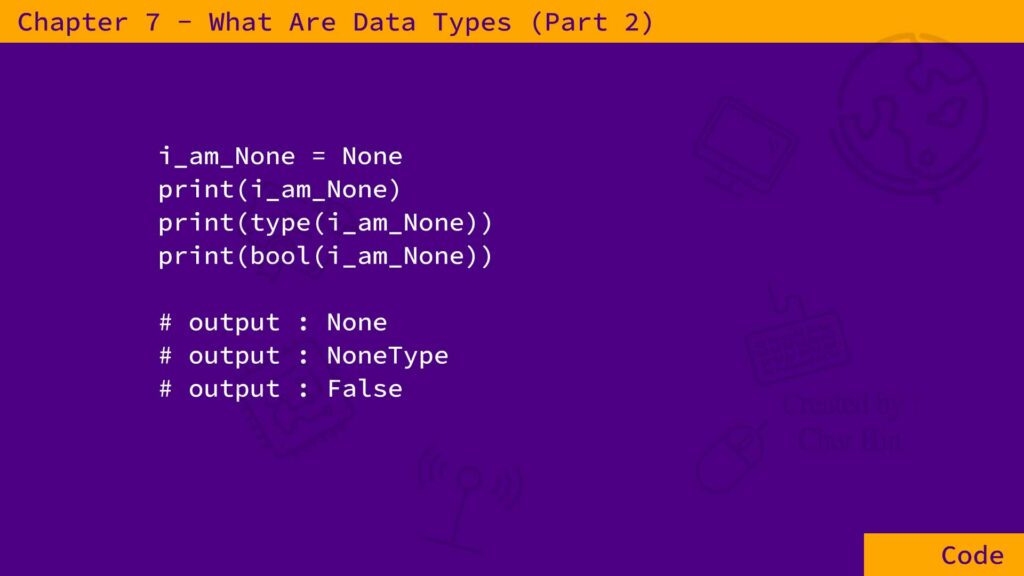
The variable i_am_None is assigned the Python keyword None.
print(i_am_None) will print None.
print(type(i_am_None)) will print NoneType.
Is None a True or False value?
print(bool(i_am_None)) will print False.
Watch my online Python course for a different learning experience.