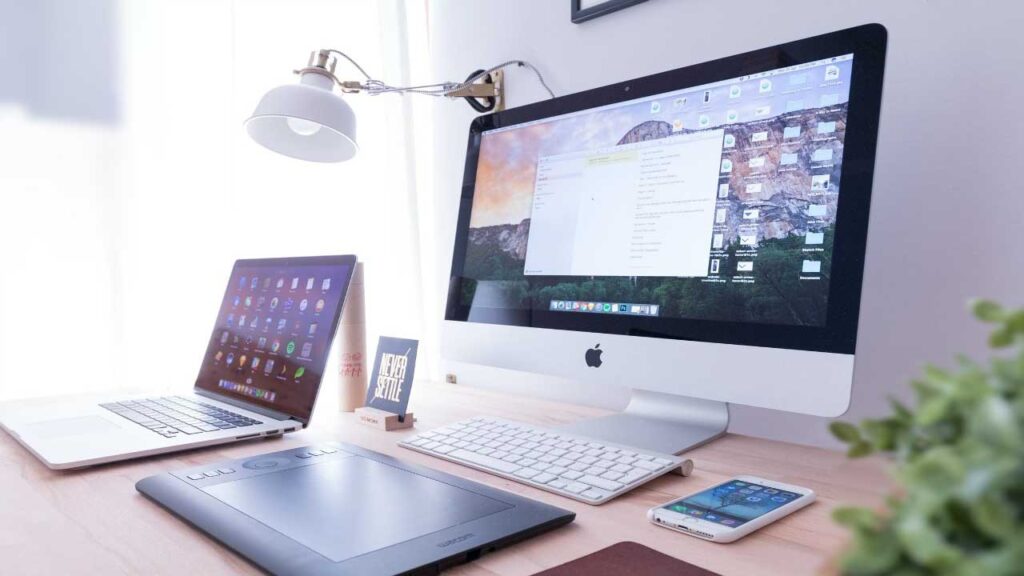
Python Tutorial 4
First Coding Lesson In Python
In this tutorial, we will start our first coding lesson.
We will cover the very basic concepts of Python — starting with the print() function, and thereafter we will cover “what are comment lines”.
Without further ado, let’s get started!
Our first program prints the words “Hello World” onto the screen.
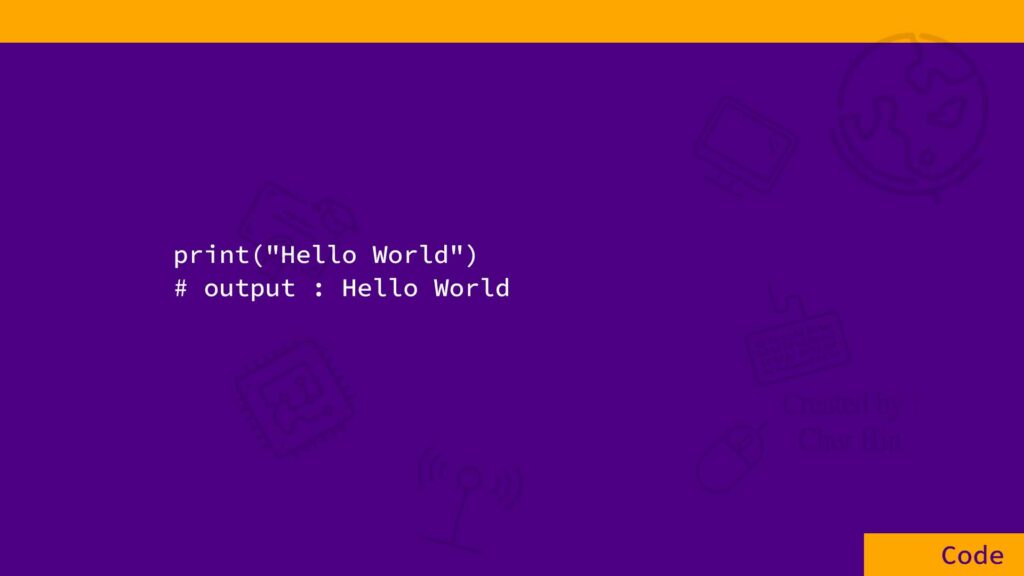
print() is a function in Python.
It prints to the screen.
Hello World
More specifically, it is a Python built-in function.
That means it comes together with the Python programming language and it’s not any external modules that we need to import before we can use it.
Functions in Python are identified by the command followed by an open parenthesis and then a close parenthesis.
Between the 2 parentheses are argument (or arguments) that we want to pass to the function.
For the print() function, it will print out whatever is between the open and close double quotation marks — in this case “Hello World”.
Single And Double Quotation Marks In Python
Take note that in Python, we can enclose “Hello World” with either single or double quotation marks.
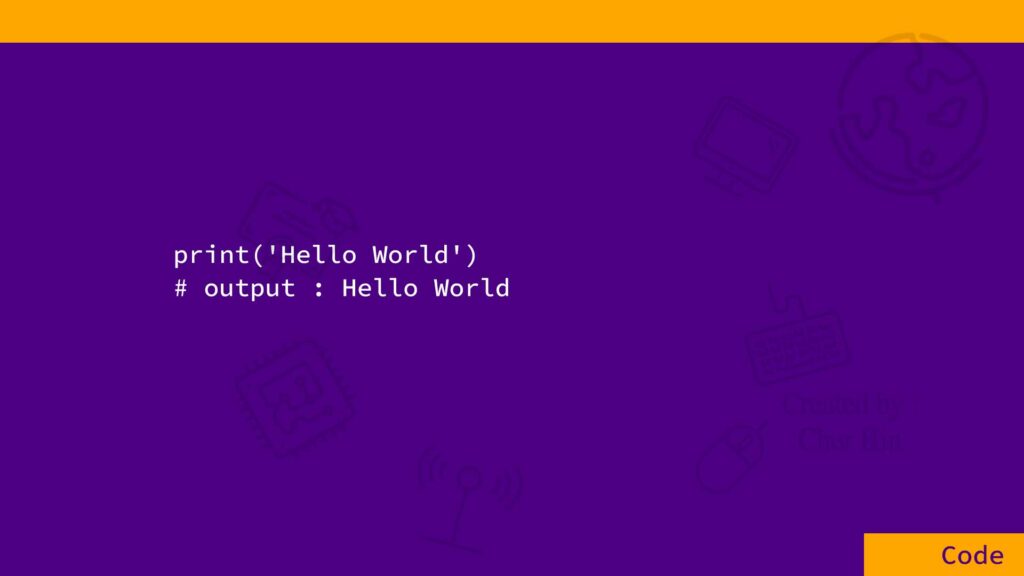
print(‘Hello World’) using single quotation marks produces the same result on the screen.
It is good programming practice to be consistent in our coding syntax.
If we use double quotes to enclose a string literal, we should use double quotes throughout our program.
In the same way, if we use single quotes to enclose a string literal, we should continue to use single quotes in the rest of our program.
That’s our first single-line program!
We will cover more about functions in future tutorials.
What Are Comments In Python
How To Write Comments In Python
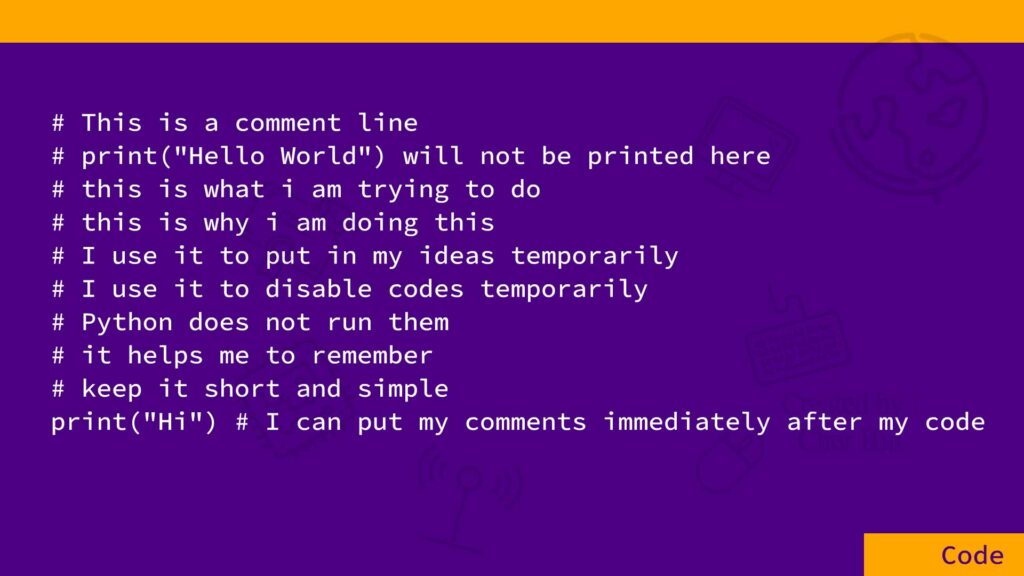
Comments in Python start with the hash character.
Comments are used to describe in plain English what our code is trying to do.
It’s like taking notes – explaining what is on our mind when we put in that very piece of code.
We could also use it to put in our ideas temporarily so that we can come back to them later on and be reminded of our ideas.
Comments are also used to disable any section of our code, for example, to remove them only temporarily for testing purposes so that we can easily “un-remove” or put back our code later on, thereby returning to our original code.
Python does not run them because they are there for descriptive purposes — they are there only for us human beings and Python ignores them.
Well-written comments help us immensely in our debugging task as it saves us a lot of time and effort trying to remember what we are trying to achieve in our piece of code.
It’s important to write clear codes.
It’s also important to write clear comments.
Keep comments short and simple to understand.
We can put comments immediately after our code.
How To Write Multiple Lines Comments In Python
What happens if we want to put in comments that span multiple lines?
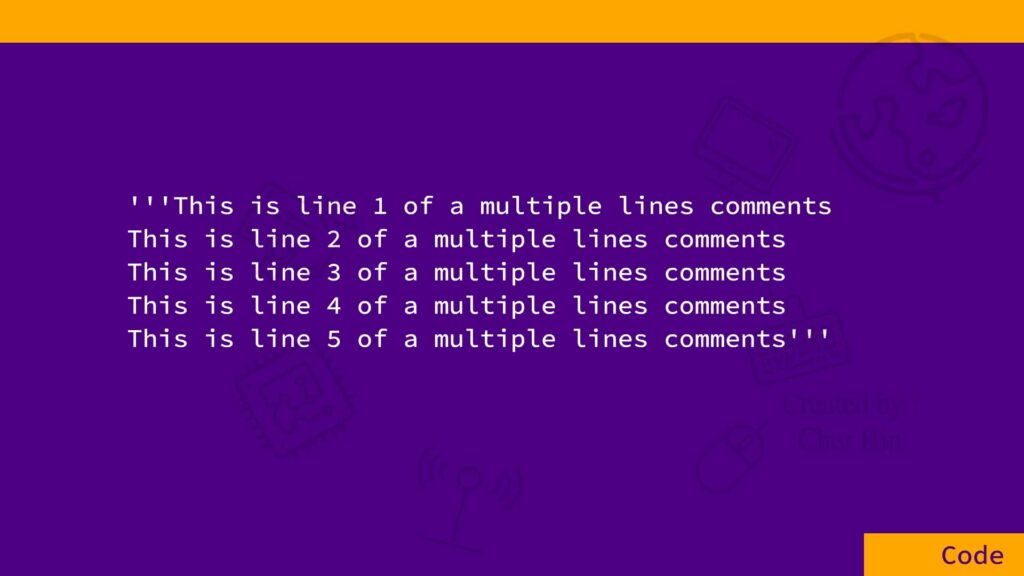
We can do so by enclosing our comment lines starting with 3 single quotation marks and ending with 3 single quotation marks.
We can also use 3 double quotation marks instead of 3 single quotation marks.
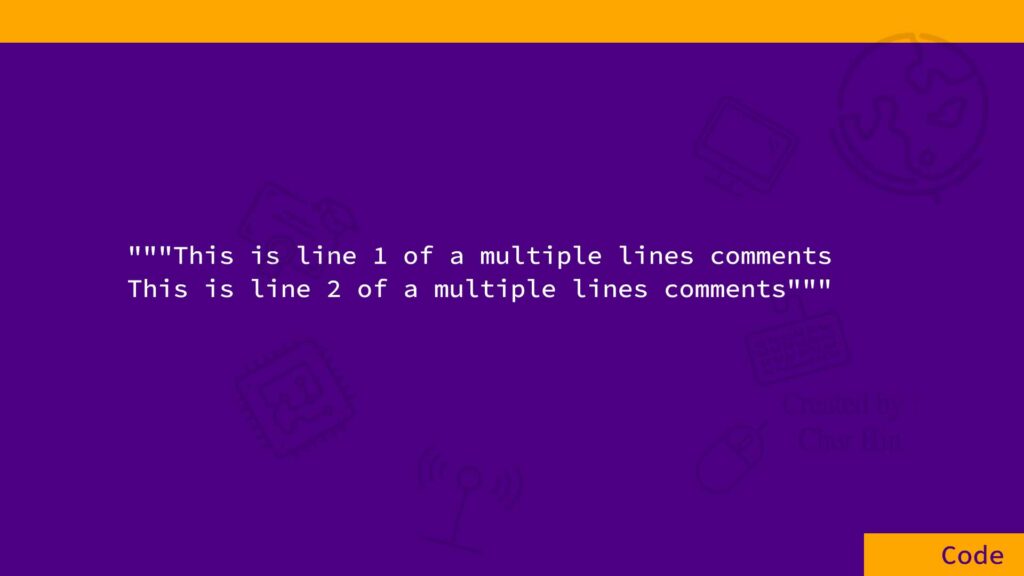
Watch my online Python course for a different learning experience.